KING88 – Truy Cập KING88 COM Nhận Liền KM 88.8K Hấp Dẫn!
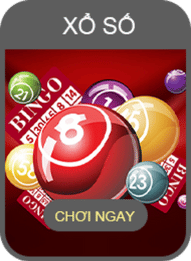
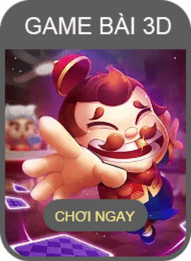
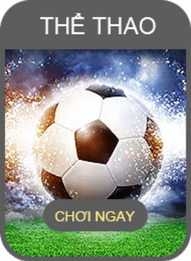
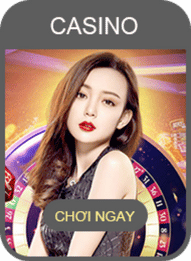
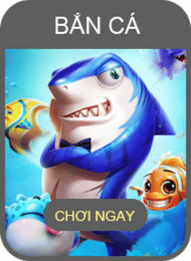
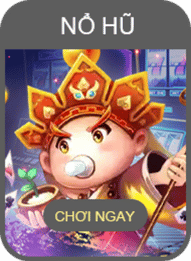
KING88 là một nhà cái uy tín, cung cấp các trò chơi cá cược đa dạng từ thể thao, casino đến game slot. Với giao diện thân thiện, khuyến mãi hấp dẫn và dịch vụ hỗ trợ 24/7, KING88 mang đến trải nghiệm đỉnh cao cho người chơi.